How to Test and Debug When You Develop an App for Shopify?
- Kishan Mehta
- Jul 25, 2024
- 6 min read
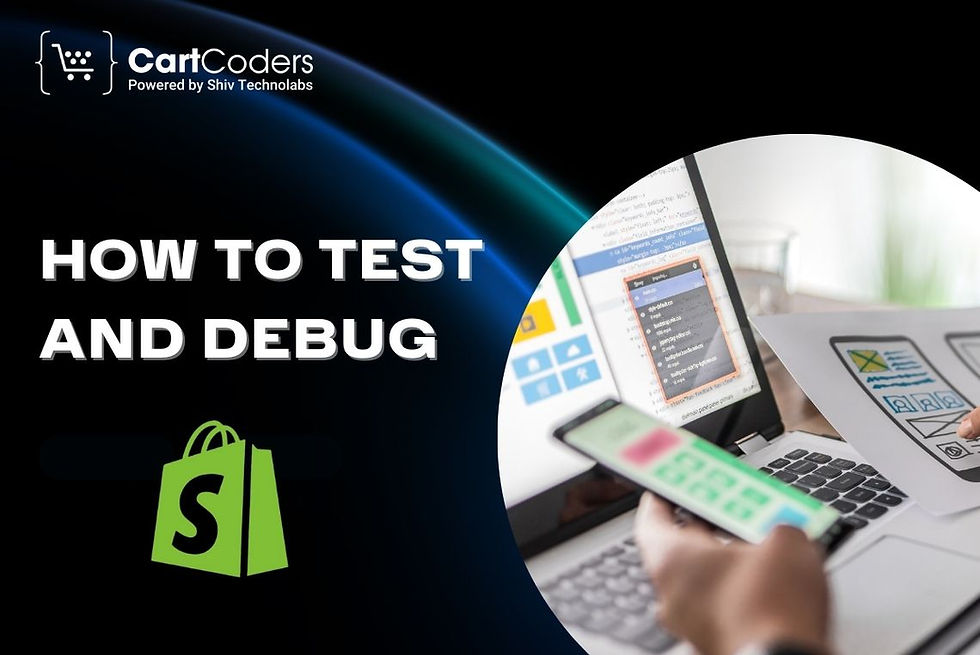
In 2024, Shopify will continue to be a leading platform for eCommerce, with over 1.75 million businesses using it worldwide. When learning how to develop an app for Shopify, testing, and debugging are critical steps to ensure your app runs smoothly and provides a great user experience. This guide will walk you through the essential aspects of testing and debugging in Shopify app development.
Setting Up Your Development Environment
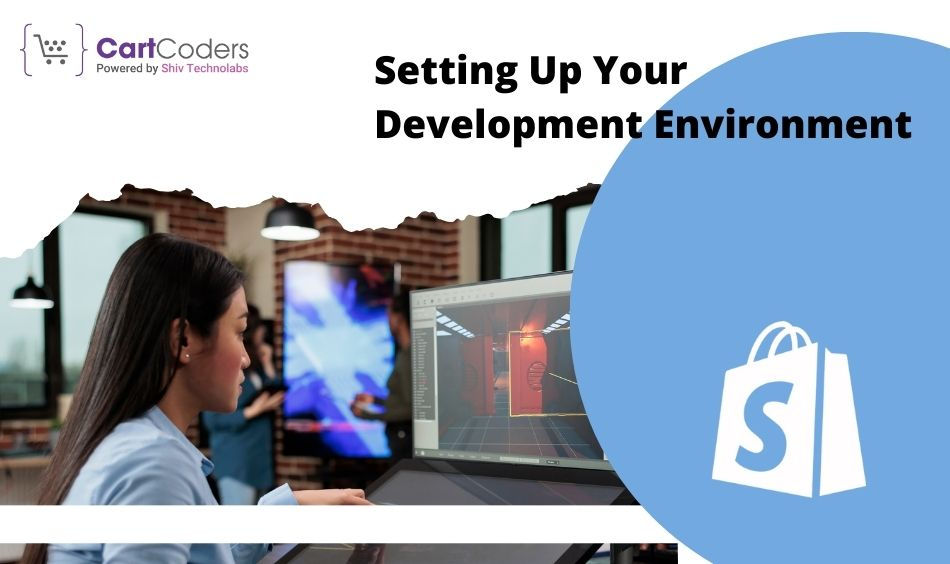
Before you start testing and debugging, it's important to set up your development environment correctly.
Tools and Resources Needed:
Shopify Partner Account: Sign up for a Shopify Partner account to get access to all necessary tools and resources.
Shopify CLI: Install Shopify CLI to assist in the development process.
Text Editor: Use a text editor like VS Code or Sublime Text to write code.
Version Control: Git for version control to track changes and collaborate with other developers.
Configuring Your Development Setup:
Create a Development Store: Use your Shopify Partner account to create a development store.
Install Shopify CLI: Follow the instructions on the Shopify documentation to install and configure Shopify CLI.
Set Up Git: Initialize a Git repository in your project directory to keep track of your changes.
bash
# Install Shopify CLI
npm install -g @shopify/cli
# Create a new Shopify app
shopify app create
# Initialize a Git repository
git init
git add .
git commit -m "Initial commit"
Writing Testable Code

Writing testable code is crucial for efficient testing and debugging.
Best Practices for Writing Clean and Testable Code:
Modular Code: Break down your code into smaller, reusable modules.
Consistent Naming Conventions: Use clear and consistent naming conventions for variables and functions.
Comments and Documentation: Add comments and documentation to explain the purpose of your code.
Organizing Your Code for Better Testing:
Follow MVC Pattern: Use the Model-View-Controller (MVC) pattern to separate concerns and make your code easier to test.
Avoid Hardcoding Values: Use configuration files or environment variables instead of hardcoding values.
javascript
// Example of a modular function
function calculateTotalPrice(items) {
return items.reduce((total, item) => total + item.price, 0);
}
// Example of a clear and consistent naming convention
const totalPrice = calculateTotalPrice(cartItems);
// Using environment variables
const API_KEY = process.env.SHOPIFY_API_KEY;
Unit Testing Your Shopify App
Unit testing helps you verify that individual components of your app work as expected.
Introduction to Unit Testing:
Definition: Unit testing involves testing individual units of code in isolation.
Importance: It helps identify bugs early in the development process.
Tools for Unit Testing in Shopify App Development:
Jest: A popular JavaScript testing framework.
Mocha: Another widely used JavaScript test framework.
Writing and Running Unit Tests:
Set Up Your Test Environment: Install Jest or Mocha and configure your test environment.
Write Test Cases: Create test cases for individual functions and components.
Run Tests: Use the testing framework’s command-line tools to run your tests and check the results.
bash
# Install Jest
npm install --save-dev jest
# Create a test script in package.json
"scripts": {
"test": "jest"
}
# Example test case using Jest
test('calculates total price of items', () => {
const items = [{ price: 10 }, { price: 20 }, { price: 30 }];
expect(calculateTotalPrice(items)).toBe(60);
});
# Run tests
npm test
Integration Testing
Integration testing verifies that different modules or services work together.
Understanding Integration Testing:
Definition: Integration testing focuses on the interaction between different units or modules.
Importance: It helps catch issues that may arise when different parts of the app interact.
Tools and Techniques for Integration Testing:
Postman: Use Postman to test API endpoints and interactions.
Supertest: A library for testing HTTP servers.
Performing Integration Tests on Shopify Apps:
Set Up Test Scenarios: Create scenarios that mimic real-world interactions between different modules.
Run Integration Tests: Execute your tests and validate the interactions and data flow between modules.
javascript
// Example integration test using Supertest
const request = require('supertest');
const app = require('./app'); // Your Express app
describe('GET /products', () => {
it('should fetch all products', async () => {
const response = await request(app).get('/products');
expect(response.status).toBe(200);
expect(response.body).toHaveLength(10); // Assuming there are 10 products
});
});
End-to-End Testing
End-to-end testing ensures that the entire application works as intended from start to finish.
What is End-to-End Testing?
Definition: End-to-end testing involves testing the complete application workflow.
Importance: It validates the entire system, including UI and backend components.
Setting Up End-to-End Testing for Shopify Apps:
Select a Framework: Use tools like Cypress or Selenium for end-to-end testing.
Define Test Scenarios: Create test scenarios that cover all critical user flows.
Tools and Frameworks for End-to-End Testing:
Cypress: An all-in-one testing framework that allows you to write and run end-to-end tests.
Selenium: A tool for automating web browsers and testing web applications.
Debugging Common Issues
Debugging is an essential part of the development process.
Identifying Common Issues in Shopify App Development:
Authentication Errors: Problems with API authentication or access tokens.
Data Sync Issues: Issues with synchronizing data between Shopify and your app.
Performance Problems: Slow load times or unresponsive features.
Effective Debugging Techniques:
Console Logging: Use console.log to print variable values and track code execution.
Debuggers: Use built-in debuggers in your text editor or browser to step through code and inspect variables.
Error Messages: Pay close attention to error messages and stack traces to pinpoint the source of issues.
Using Shopify's Built-in Debugging Tools:
Shopify App Bridge: A tool for debugging embedded apps.
GraphQL Explorer: Use the GraphQL Explorer to test and debug API queries.
Monitoring and Logging
Monitoring and logging help you track the performance and health of your app.
Importance of Monitoring and Logging:
Performance Monitoring: Track the performance of your app and identify bottlenecks.
Error Logging: Log errors and exceptions to troubleshoot issues.
Tools for Monitoring Your Shopify App:
New Relic: A tool for performance monitoring and analysis.
Loggly: A cloud-based log management service.
Setting Up Logging for Better Debugging:
Implement Logging: Add logging statements to your code to record important events and errors.
Monitor Logs: Regularly review logs to identify and resolve issues.
Using Shopify's Built-in Tools
Shopify provides several tools to help with testing and debugging.
Overview of Shopify's Debugging Tools:
Shopify CLI: A command-line tool for managing Shopify apps.
GraphQL Explorer: A tool for exploring and testing GraphQL queries.
Utilizing Shopify CLI for Testing and Debugging:
Commands: Use Shopify CLI commands to manage and test your app.
App Deployment: Deploy your app to a development store for testing.
Leveraging Shopify Partners Dashboard:
App Management: Use the Partners Dashboard to manage your app settings and monitor usage.
Error Reporting: View error reports and logs to identify issues.
Continuous Integration and Continuous Deployment (CI/CD)
CI/CD automates the testing and deployment process.
Understanding CI/CD in Shopify App Development:
Definition: Continuous Integration (CI) involves automatically testing code changes, while Continuous Deployment (CD) involves automatically deploying those changes.
Setting Up CI/CD Pipelines:
Choose a CI/CD Tool: Use tools like Jenkins, GitHub Actions, or GitLab CI.
Configure Pipelines: Set up pipelines to automatically test and deploy your app.
Best Practices for CI/CD in Shopify Apps:
Automate Testing: Ensure that all tests run automatically with each code change.
Automate Deployment: Automatically deploy successful builds to a development store.
Performance Testing
Performance testing helps you measure how well your app performs under various conditions.
Importance of Performance Testing:
User Experience: Ensure a smooth and fast user experience.
Scalability: Validate that your app can handle increased load.
Tools for Performance Testing in Shopify:
JMeter: A tool for load testing and measuring performance.
Lighthouse: A tool for auditing performance, accessibility, and SEO.
Conducting Performance Tests:
Define Test Scenarios: Create scenarios to test different aspects of performance.
Run Tests: Execute tests and analyze the results to identify performance issues.
Security Testing
Security testing ensures that your app is secure from vulnerabilities.
Overview of Security Testing:
Definition: Security testing involves identifying and fixing security vulnerabilities in your app.
Importance: Protects user data and ensures compliance with regulations.
Common Security Issues in Shopify Apps:
Cross-Site Scripting (XSS): Injecting malicious scripts into web pages.
SQL Injection: Injecting malicious SQL code into queries.
Authentication Flaws: Weak authentication mechanisms.
Tools and Techniques for Security Testing:
OWASP ZAP: An open-source security testing tool.
Burp Suite: A comprehensive security testing platform.
Conclusion
Testing and debugging are vital components of Shopify app development. By following best practices and using the right tools, you can build reliable, high-quality apps that provide a great user experience. Always remember to write testable code, use automated testing, and monitor performance and security to ensure your app's success.
For businesses looking to develop a custom Shopify app, partnering with a professional Shopify app development company like CartCoders can make all the difference in achieving a smooth and efficient development process.
Comments